All tools in the toolbox can be customized using system defined tool
events and executed by user defined Python scripts.
The scripts are available
from the General tab of all tools.
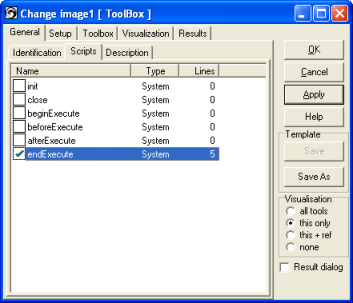
The events are called by the
tool list object. This is a very powerful feature that can substantially
reduce the length of the toolbox. The script's mouse menu is shown
below:
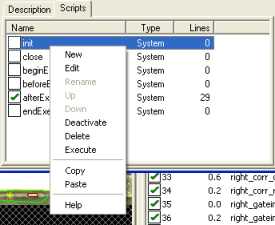
- New - creates a user defined script
- Edit - opens script editor
- Rename - renames user defined script
- Up - moves script up
- Down - moves script down
- Deactivate - deactivates selected script
- Delete - deletes script - removes user defined script | empties
system defined script
- Copy - copies script to clipboard
- Paste - pastes script from clipboard to selected script
- Help activates scripting help
How it works
When a tool activates a script, the tool list make an instance of a 'hidden'
Python class in the Python namespace for the actual tool.
The class
definition is given by the activated scripts.
This class has a member 'name' which can be used within the scripts to
get access to the python tool object
This 'hidden' class is a standard python object, you can add members and
methods as for any standard Python class by using the self argument which
always must be the first argument
- def init()
- self.mymember=10
- self.counter = 0
- self.maxCount = 12
Note: The local attributes are encapsulated and should be used to
preserve state of the between the eventhandlers
Methods |
Description |
init |
called at tool creation and when the user applies changes to any
script. Useful to customize the python tool instance. The init
method is paired with the close method. |
close |
called at tool destruction and when the user applies changes to
any script. May be used for cleanup. The close method is paired with
the init method. |
beginExecute |
called once before the actual tool execution. In this method it
is possible to write its own processing algorithms, iterate itself
or any kind of processing. By returning 1 from this method the
default tool execution will not be executed. This method is also
useful for setting up internal states and parameters before image
processing. |
beforeExecute |
called just before tool execution, also when iterating the tool
from Python. |
afterExecute |
called just after tool execution, also when iterating the tool
from Python. By returning 0 the tool will execute again until
afterExecute returns 1. Sometimes useful for result validation.
Special care should be taken to avoid entering a endless loop (by
always returning 0) |
endExecute |
called at last in tool execution, enables to collect results,
cleanup etc. This method is called only once for each tool in
toolbox execution. Returning 1 from endExecute signals tool
execution error. |
Example 1: Iterate itself
def init(self): self.count=0 #define a local counter self.max=12 #define a local max count
def beginExecute(self): self.count=0 #reset counter t=GetTool(self.name) #get
the python tool instance for myself img=GetImageMatr('Box') #get the image to process ResetStatistics() #userdefined method for statistics for i in range(self.max): SetROI() #userdefined method for settng ROI t.execute(img) #execute the tool at new location UpdateStatistics() #userdefined method for collect results and update statistics return 1 #abort default processing/execution
Example 2: Set Value of a ExternalLogic using business logic
def afterExecute(self):
#use self.name to get this tool instance
#tool=GetTool(self.name)
#Return 1 for OK, 0 for new execution
type = GetIntValue('Type.Value')
fitFraction = GetValue('CircleFitFraction.Value')
circleLocated = 0
if type == 1:
circleLocated = GetIntValue('RadiusType1-3.FitFraction') > fitFraction
if type == 2:
circleLocated = GetIntValue('RadiusType2-3.FitFraction') > fitFraction
if type == 3:
circleLocated = GetIntValue('RadiusType3-3.FitFraction') > fitFraction
SetBoolValue(self.name,circleLocated)
return 1
Example 3: Iterate a RadialArcFinder in N posistion to find the best fitFraction
# define local attributes
def init(self):
self.counter = 0
self.bestFit = 0
self.bestX = 0.0
# reset the local attributes for each tool execution
def beginExecute(self):
#use self.name to get this tool instance
#tool=GetTool(self.name)
#Return 1 if handled by script, 0 for default handling
self.counter = 0
self.bestFit = 0
self.bestX = 0
return 0
# state model in afterExecute
# iterate N position to locate best fitFraction
# rerun the the tool for the best fitFraction
def afterExecute(self):
#use self.name to get this tool instance
#tool=GetTool(self.name)
#Return 1 for OK, 0 for new execution
iterations = 3
tool = GetTool(self.name)
fit = tool.getIntValue('FitFraction')
if self.counter < iterations:
tool.clearOverlay()
self.counter = self.counter + 1
print ' radius center type 3 - fit ',fit,self.counter
point = GetTool('CenterType3')
if fit > self.bestFit:
self.bestFit = fit
self.bestX = point.getIntValue('Value_x')
point.setValue('Value_x',point.getIntValue('Value_x')-75)
img = GetImageMatr('Bilde')
point.execute(img)
return 0
if self.counter == iterations :
tool.clearOverlay()
self.counter = self.counter + 1
point = GetTool('CenterType3')
point.setValue('Value_x',self.bestX)
img = GetImageMatr('Bilde')
point.execute(img)
return 0
if self.counter == iterations +1 :
return 1
|