More complex
OverlayPanel with WinForms controls example
This example is created to show how to use some
of the more popular controls in the .NET Windows.Forms namespace.
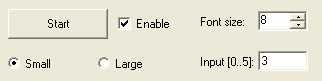
Here is the GUI created by the following script
example.
This is parts of the Dotnet - Controls example
contained on the Scorpion CD.
#
Central Start Script
profile = GetStringValue('System.Profile')
print '---------------------------'
print GetCurSource()
RegisterPlugin(CreateResultPanel(GetResultPanel().handle))
RegisterPlugin(CreateOpenCentralOverlay(GetCustomPage('Demo').handle))
RegisterPlugin(CreateTeachOverlay(GetPanel('customPanel1').handle))
RegisterPlugin(CreateImageViewConnection())
#
CreateResultPanel method
def CreateResultPanel(hwnd):
import CLR
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, ContentAlignment, Font
from CLR.System import Int32, Decimal
# Load custom assemblies
from CLR.System.Reflection import Assembly
Assembly.LoadFrom('OverlayPanel.dll')
from CLR.Tordivel import OverlayPanel
# Custom .NET components should be placed in DotNet folder in profile path
class ResultPanelOverlay(OverlayPanel):
def __init__(self,nativeParentHandleAsInt32):
# This enables the panel to follow resizing of the parent
self.Dock = WinForms.DockStyle.Fill
# Create the errorProvider control
self.errorProvider = WinForms.ErrorProvider()
self.errorProvider.BlinkStyle = WinForms.ErrorBlinkStyle.NeverBlink
# Create the button
self.button = WinForms.Button()
self.button.Parent = self
self.button.Size = Size(100,30)
self.button.Location = Point(10,10)
self.button.Text = "Start"
# Register event handler for button.Click
self.button.Click += self.button_ClickHandler
# Create the checkBox
self.checkBox = WinForms.CheckBox()
self.checkBox.Parent = self
self.checkBox.Size = Size(80,30)
self.checkBox.Location = Point(120,10)
self.checkBox.Text = "Enable"
self.checkBox.Checked = 1
# Register event handler for checkBox.CheckedChanged
self.checkBox.CheckedChanged += self.checkBox_CheckedChangedHandler
# Create the numericUpDown
self.numericUpDown = WinForms.NumericUpDown()
self.numericUpDown.Parent = self
self.numericUpDown.Size = Size(50,25)
self.numericUpDown.Location = Point(260,12)
self.numericUpDown.Minimum = Decimal(8)
self.numericUpDown.Maximum = Decimal(20)
self.numericUpDown.Value = Decimal(11)
# Register event handler for numericUpDown.ValueChanged
self.numericUpDown.ValueChanged += self.numericUpDown_ValueChangedHandler
# Create the label (after the numericupdown to avoid hiding)
self.label = WinForms.Label()
self.label.Parent = self
self.label.Size = Size(70,25)
self.label.Location = Point(200,10)
self.label.Text = "Font size:"
self.label.TextAlign = ContentAlignment.MiddleLeft
# Create the smallRadioButton
self.smallRadioButton = WinForms.RadioButton()
self.smallRadioButton.Parent = self
self.smallRadioButton.Size = Size(60,30)
self.smallRadioButton.Location = Point(10,50)
self.smallRadioButton.Text = "Small"
# Register event handler for smallRadioButton.CheckedChanged
self.smallRadioButton.CheckedChanged += self.smallRadioButton_CheckedChangedHandler
# Create the largeRadioButton
self.largeRadioButton = WinForms.RadioButton()
self.largeRadioButton.Parent = self
self.largeRadioButton.Size = Size(60,30)
self.largeRadioButton.Location = Point(100,50)
self.largeRadioButton.Text = "Large"
# Register event handler for largeRadioButton.CheckedChanged
self.largeRadioButton.CheckedChanged += self.largeRadioButton_CheckedChangedHandler
# Create the inputTextBox
self.inputTextBox = WinForms.TextBox()
self.inputTextBox.Parent = self
self.inputTextBox.Size = Size(50,25)
self.inputTextBox.Location = Point(260,52)
self.inputTextBox.Text = "3"
# Register event handler for inputTextBox.TextChanged
self.inputTextBox.TextChanged += self.inputTextBox_TextChangedHandler
# Create the inputLabel (after the textBox to avoid hiding)
self.inputLabel = WinForms.Label()
self.inputLabel.Parent = self
self.inputLabel.Size = Size(100,25)
self.inputLabel.Location = Point(200,50)
self.inputLabel.Text = "Input [0..5]:"
self.inputLabel.TextAlign = ContentAlignment.MiddleLeft
def button_ClickHandler(self, sender, args):
# Handles the button Click event
if self.button.Text == "Start":
self.button.Text = "Stop"
elif self.button.Text == "Stop":
self.button.Text = "Start"
def checkBox_CheckedChangedHandler(self, sender, args):
# Handles the checkBox CheckedChanged event
if self.checkBox.Checked == 1:
self.button.Enabled = 1
elif self.checkBox.Checked == 0:
self.button.Enabled = 0
def numericUpDown_ValueChangedHandler(self, sender, args):
# Handles the numericUpDown ValueChanged event
newFont = Font(self.button.Font.FontFamily.Name,Decimal.ToInt32(self.numericUpDown.Value))
self.button.Font = newFont
def smallRadioButton_CheckedChangedHandler(self, sender, args):
# Handles the smallRadioButton CheckedChanged event
if self.smallRadioButton.Checked == 1:
self.numericUpDown.Value = self.numericUpDown.Minimum
def largeRadioButton_CheckedChangedHandler(self, sender, args):
# Handles the largeRadioButton CheckedChanged event
if self.largeRadioButton.Checked == 1:
self.numericUpDown.Value = self.numericUpDown.Maximum
def inputTextBox_TextChangedHandler(self, sender, args):
# Handles the inputTextBox TextChanged event
self.errorProvider.SetError(self.inputTextBox,"")
try:
newValue = Int32.Parse(self.inputTextBox.Text)
if newValue < 0:
self.errorProvider.SetError(self.inputTextBox,"Values less than zero are invalid")
elif newValue > 5:
self.errorProvider.SetError(self.inputTextBox,"Values larger than 5 are invalid")
except:
self.errorProvider.SetError(self.inputTextBox,"Invalid input")
return ResultPanelOverlay(hwnd)
#
CreateImageViewConnection() method
def CreateImageViewConnection():
# pc=GetPageControl('ImageView.pc')
# ts=pc.getPage('Image')
# cp=ts.getControl('CustomPanel')
cp = GetImageView().getCustomPanel('Image')
cp.color='red'
cp.font.color='white'
import CLR
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, ContentAlignment, Font, Color
from CLR.System import Int32, Decimal
class Labels(WinForms.Panel):
def __init__(self):
self.BorderStyle = WinForms.BorderStyle.FixedSingle
def Init(self,rows,fontName,fontSize):
self.rows = rows
self.hSpace = 1.5 * fontSize
self.labels = []
# Create the label (after the numericupdown to avoid hiding)
for i0 in range(self.rows):
self.labels.append(WinForms.Label())
self.labels[i0].Parent = self
self.labels[i0].Size = Size(200,self.hSpace)
self.labels[i0].Location = Point((self.Width-self.labels[i0].Width)/2,self.hSpace*(i0+1))
self.labels[i0].Text = "Line" + str(i0)
newFont = Font(fontName,fontSize)
self.labels[i0].Font = newFont
self.labels[i0].ForeColor = Color.Green
self.labels[i0].TextAlign = ContentAlignment.MiddleCenter
# Register event handler for Resize
self.Resize += self.ResizeHandler
def ResizeHandler(self, sender, args):
# Handles the Resize event
print 'Resize Left: ',self.Left
print 'Resize Top: ',self.Top
print 'Resize Width: ',self.Width
print 'Resize Height: ',self.Height
#self.hSpace = max(200,self.Height) / (self.rows )
for i0 in range(self.rows):
self.labels[i0].Location = Point((self.Width-self.labels[i0].Width)/2,self.hSpace*(i0+1))
def SetLine(self, lineNo, text):
if 0 < lineNo <= self.rows :
self.labels[lineNo-1].Text = text
def GetLine(self, lineNo):
if 0 < lineNo <= self.rows :
text = str( self.labels[lineNo-1].Text )
return text
else:
return '-'
class ImageViewButton(WinForms.Button):
def __init__(self):
# Create the button
self.Text = "Open Central Start"
# Register event handler for button.Click
self.Click += self.button_ClickHandler
def button_ClickHandler(self, sender, args):
# Handles the button Click event
if self.Text == "Open Central Start":
self.Text = "Close Central Start"
# open service
ExecuteCmd('AccessControl','Service=1')
#activate Service
mp = GetPageControl('pcMain')
mp.activePage = 'Service'
#activate Advanced
sp = GetPageControl('pcService')
sp.activePage = 'Advanced'
# activate Central
sap = GetPageControl('pcServiceAdvanced')
sap.activePage = 'Central'
elif self.Text == "Close Central Start":
self.Text = "Open Central Start"
# open service
ExecuteCmd('AccessControl','Service=0')
mp = GetPageControl('pcMain')
mp.activePage = 'Operation'
# Load custom assemblies
from CLR.System.Reflection import Assembly
Assembly.LoadFrom('OverlayPanel.dll')
from CLR.Tordivel import OverlayPanel
class ImageViewOverlay(OverlayPanel):
def __init__(self,nativeParentHandleAsInt32):
# This enables the panel to follow resizing of the parent
self.Dock = WinForms.DockStyle.Fill
# Create the button
self.button = ImageViewButton()
self.button.Parent = self
self.button.Size = Size(100,30)
self.button.Location = Point((self.Width-self.button.Width)/2,self.Height-self.button.Height*2)
self.Labels = Labels()
self.Labels.Parent = self
self.Labels.Size = Size(self.Width,100)
self.Labels.Location = Point(0,0)
self.Labels.Init(3,'Arial',15.0)
# Register event handler for Resize
self.Resize += self.ResizeHandler
RegisterCallback('Actions.AfterInspect',self.AfterInspect)
def ResizeHandler(self, sender, args):
# Handles the Resize event
self.button.Location = Point((self.Width-self.button.Width)/2,self.Height-self.button.Height*2)
self.Labels.Size = Size(self.Width,100)
def AfterInspect(self):
ok = GetBoolValue('pm.OK')
match = GetValue('pm.FitPercent')
angle = GetValue('pm.Angle')
scaleX = GetValue('pm.ScaleX')
scaleY = GetValue('pm.ScaleY')
str0 = 'Fit: %(match).1f ok: %(ok).0f' %vars()
self.Labels.SetLine(1,str0)
str0 = 'Angle: %(angle).1f' %vars()
self.Labels.SetLine(2,str0)
str0 = 'Scale: %(scaleX).2f,%(scaleY).2f' %vars()
self.Labels.SetLine(3,str0)
return ImageViewOverlay(cp.handle)
|