Using the OwnedForm Tordivel .Net Object, it is easy to implement
custom modeless and modal dialogs. The example below shows how to
implement a class derived from OwnedForm again derived from the Form
class - used to add a modeless dialog containing a Button.
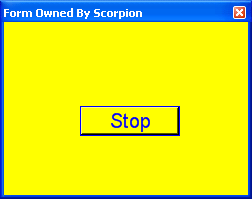
import CLR
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, Color, Font
# Load custom assemblies
from CLR.System.Reflection import Assembly
scorpionDir = GetValue('System.ScorpionDir')
Assembly.LoadFrom(scorpionDir + r'\OverlayPanel.dll')
from CLR.Tordivel import OverlayPanel
# Custom .NET components should be placed in DotNet folder in profile
path
profile = GetValue('System.Profile')
Assembly.LoadFrom(profile + r'\DotNet\OwnedForm.dll')
from CLR.Tordivel import OwnedForm
class ScorpionForm(OwnedForm):
def __init__(self,nativeParentHandleAsInt32):
self.Text = "Form Owned By Scorpion"
self.MaximizeBox = 0
self.MinimizeBox = 0
self.ShowInTaskbar = 0
self.FormBorderStyle = WinForms.FormBorderStyle.SizableToolWindow
self.BackColor = Color.Yellow;
self.ForeColor = Color.Blue;
self.Font = Font('Arial',15.0)
self.Closing += self.ClosingHandler
self.Size = Size(400,300)
# Create the button
self.button = WinForms.Button()
self.button.Parent = self
self.button.Size = Size(100,30)
self.button.Location = Point((self.Width-self.button.Width)/2,(self.Height-self.button.Height)/2)
self.button.Text = "Start"
# Register event handler for button.Click
self.button.Click += self.button_ClickHandler
# Register event handler for Resize
self.Resize += self.ResizeHandler
def button_ClickHandler(self, sender, args):
# Handles the button Click event
if self.button.Text == "Start":
self.button.Text = "Stop"
elif self.button.Text == "Stop":
self.button.Text = "Start"
def ResizeHandler(self, sender, args):
# Handles the Resize event
#print 'ResizeHandler'
self.button.Location = Point((self.Width-self.button.Width)/2,(self.Height-self.button.Height)/2)
def ClosingHandler(self, sender, args):
# Handles the Resize event
args.Cancel = 1 # deny close request
class ResultPanelOverlay(OverlayPanel):
def __init__(self,nativeParentHandleAsInt32):
# This enables the panel to follow resizing of the parent
self.Dock = WinForms.DockStyle.Fill
self.scorpionForm = None
# Create the button
self.button = WinForms.Button()
self.button.Parent = self
self.button.Size = Size(100,30)
self.button.Location = Point(10,10)
self.button.Text = "Show"
# Register event handler for button.Click
self.button.Click += self.button_ClickHandler
# Create the button
self.hbutton = WinForms.Button()
self.hbutton.Parent = self
self.hbutton.Size = Size(100,30)
self.hbutton.Location = Point(10,10)
self.hbutton.Text = "Hide"
# Register event handler for button.Click
self.hbutton.Click += self.hbutton_ClickHandler
# Register event handler for Resize
self.Resize += self.ResizeHandler
def button_ClickHandler(self, sender, args):
# Handles the button Click event
if self.scorpionForm == None:
print 'Create ScorpionForm'
self.scorpionForm =
ScorpionForm(GetResultPanel().handle)
# register ClosedHandler to detect when
recreating is necessary
self.scorpionForm.Closed +=
self.ScorpionForm_ClosedHandler
self.scorpionForm.Show()
else:
print 'Activate ScorpionForm'
self.scorpionForm.Show()
self.scorpionForm.Activate()
def hbutton_ClickHandler(self, sender, args):
# Handles the button Click event
if self.scorpionForm == None:
print 'Create ScorpionForm'
self.scorpionForm =
ScorpionForm(GetResultPanel().handle)
# register ClosedHandler to detect when
recreating is necessary
self.scorpionForm.Closed +=
self.ScorpionForm_ClosedHandler
self.scorpionForm.Hide()
else:
print 'Hide'
self.scorpionForm.Hide()
def ScorpionForm_ClosedHandler(self, sender, args):
# Handles the Closed event
print 'ClosedHandler'
self.scorpionForm = None
def ResizeHandler(self, sender, args):
# Handles the Resize event
print 'ResizeHandler'
self.button.Location = Point((self.Width-self.button.Width)/2,(self.Height-self.button.Height)/2)
self.hbutton.Location = Point((self.Width-self.button.Width)/2-120,(self.Height-self.button.Height)/2)
# Dispose existing version of resultPanelOverlay
try:
resultPanelOverlay.Dispose()
except:
print 'Dispose Failed'
# Create global instance of resultPanelOverlay
resultPanelOverlay = ResultPanelOverlay(GetResultPanel().handle) |