This example simulates guiding an operator
through some sequence of operations.
The script depends on the Tordivel.OverlayPanel
component and a JPEG image named "Water lilies.jpg". In addition a
custom panel named "Status" must be created to host the
Tordivel.OverlayPanel component.
A simple timer is used to drive the example
using a fixed interval of 5 seconds.
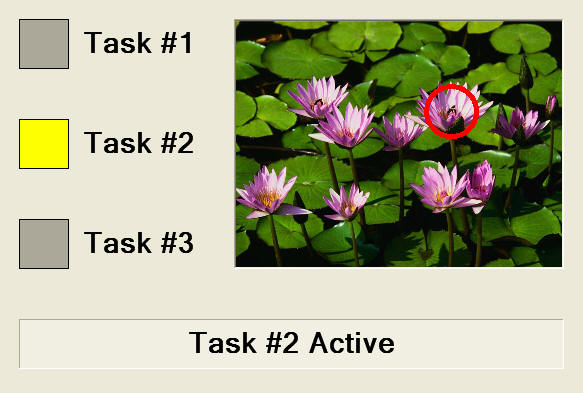
# Central Start
# Python start script goes here
print 'Central Start'
statusPanel = CreateStatusPanel(GetCustomPage('Status').handle)
# Central Stop
print 'Central Stop'
try:
statusPanel.Dispose()
print 'dispose statusPanel'
except:
pass
def CreateStatusPanel
def CreateStatusPanel(hwnd):
import CLR
from CLR.System import Random
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, Pen, Color, SystemColors
from CLR.System.Drawing import ContentAlignment, Font, FontStyle, Image
# Load custom assemblies
from CLR.System.Reflection import Assembly
Assembly.LoadFrom('OverlayPanel.dll')
from CLR.Tordivel import OverlayPanel
profile = GetValue('System.Profile')
# define StatusPanel class by inheriting from OverlayPanel
class StatusPanel(OverlayPanel):
def __init__(self,nativeParentHandleAsInt32):
self.Dock = WinForms.DockStyle.Fill
self.TaskPanel1 = WinForms.Panel()
self.TaskPanel1.Parent = self
self.TaskPanel1.BorderStyle = WinForms.BorderStyle.FixedSingle
self.TaskPanel1.Size = Size(50, 50)
self.TaskPanel2 = WinForms.Panel()
self.TaskPanel2.Parent = self
self.TaskPanel2.BorderStyle = WinForms.BorderStyle.FixedSingle
self.TaskPanel2.Size = Size(50, 50)
self.TaskPanel3 = WinForms.Panel()
self.TaskPanel3.Parent = self
self.TaskPanel3.BorderStyle = WinForms.BorderStyle.FixedSingle
self.TaskPanel3.Size = Size(50, 50)
self.TaskLabel1 = WinForms.Label()
self.TaskLabel1.Parent = self
self.TaskLabel1.BorderStyle = WinForms.BorderStyle.None
self.TaskLabel1.Font = Font("Microsoft Sans Serif", 21.75, FontStyle.Bold)
self.TaskLabel1.Size = Size(150, 50)
self.TaskLabel1.Text = "Task #1"
self.TaskLabel1.TextAlign = ContentAlignment.MiddleLeft
self.TaskLabel2 = WinForms.Label()
self.TaskLabel2.Parent = self
self.TaskLabel2.BorderStyle = WinForms.BorderStyle.None
self.TaskLabel2.Font = Font("Microsoft Sans Serif", 21.75, FontStyle.Bold)
self.TaskLabel2.Size = Size(150, 50)
self.TaskLabel2.Text = "Task #2"
self.TaskLabel2.TextAlign = ContentAlignment.MiddleLeft
self.TaskLabel3 = WinForms.Label()
self.TaskLabel3.Parent = self
self.TaskLabel3.BorderStyle = WinForms.BorderStyle.None
self.TaskLabel3.Font = Font("Microsoft Sans Serif", 21.75, FontStyle.Bold)
self.TaskLabel3.Size = Size(150, 50)
self.TaskLabel3.Text = "Task #3"
self.TaskLabel3.TextAlign = ContentAlignment.MiddleLeft
self.PictureBox = WinForms.PictureBox()
self.PictureBox.Parent = self
self.PictureBox.BorderStyle = WinForms.BorderStyle.Fixed3D
self.PictureBox.SizeMode = WinForms.PictureBoxSizeMode.StretchImage
self.PictureBox.Image = Image.FromFile(profile + r'\DotNet\Water lilies.jpg')
self.PictureBox.Size = Size(330, 250)
self.PictureBox.Paint += self.PictureBoxPaintHandler
self.InfoLabel = WinForms.Label()
self.InfoLabel.Parent = self
self.InfoLabel.BackColor = SystemColors.ControlLight
self.InfoLabel.BorderStyle = WinForms.BorderStyle.Fixed3D
self.InfoLabel.Font = Font("Microsoft Sans Serif", 21.75, FontStyle.Bold)
self.InfoLabel.Size = Size(400, 50)
self.InfoLabel.Text = "Information"
self.InfoLabel.TextAlign = ContentAlignment.MiddleCenter
self.UpdateLayout()
self.TaskNumber = 1
self.TaskState = 'Active'
self.RedCircleLocationX = -1
self.RedCircleLocationY = -1
self.UpdateView()
# Create timer and register tick eventhandler
self.timer = WinForms.Timer()
self.Components.Add(self.timer)
self.timer.Tick += self.TimerTickHandler
self.timer.Interval = 5000
self.timer.Enabled = 1
# Register event handler for Resize
self.Resize += self.ResizeHandler
def PictureBoxPaintHandler(self, sender, args):
# Handle picture box paint
#print 'PictureBoxPaintHandler'
if self.RedCircleLocationX >= 0:
args.Graphics.DrawEllipse(Pen(Color.Red,5.0),self.RedCircleLocationX,self.RedCircleLocationY,50,50);
def UpdateLayout(self):
# Update control positions and size
self.TaskPanel1.Location = Point(20,20);
self.TaskPanel2.Location = Point(20,120);
self.TaskPanel3.Location = Point(20,220);
self.TaskLabel1.Location = Point(80,20);
self.TaskLabel2.Location = Point(80,120);
self.TaskLabel3.Location = Point(80,220);
self.PictureBox.Location = Point(self.Width-(self.PictureBox.Width+20), 20)
self.InfoLabel.Location = Point(20,320);
self.InfoLabel.Size = Size(self.Width-40,50);
def UpdateState(self):
# Simulate internal state change
#print 'UpdateState TaskNumber:',self.TaskNumber,' TaskState:',self.TaskState
if self.TaskState != 'Active':
self.TaskNumber += 1
if self.TaskNumber > 3:
self.TaskNumber = 1
self.TaskState = 'Active'
else:
random = Random()
if random.Next(100) < 90:
self.TaskState = 'Succeded'
else:
self.TaskState = 'Failed'
self.UpdateView()
def UpdateView(self):
# Updates view according to internal state
#print 'UpdateView'
if self.TaskNumber == 1:
if self.TaskState == 'Active':
self.TaskPanel1.BackColor = Color.Yellow
self.InfoLabel.Text = "Task #1 Active"
elif self.TaskState == 'Succeded':
self.TaskPanel1.BackColor = Color.Green
self.InfoLabel.Text = "Task #1 Succeded"
elif self.TaskState == 'Failed':
self.TaskPanel1.BackColor = Color.Red
self.InfoLabel.Text = "Task #1 Failed"
else:
self.TaskPanel1.BackColor = SystemColors.ControlDark
self.RedCircleLocationX = 60
self.RedCircleLocationY = 50
self.PictureBox.Invalidate()
else:
self.TaskPanel1.BackColor = SystemColors.ControlDark
if self.TaskNumber == 2:
if self.TaskState == 'Active':
self.TaskPanel2.BackColor = Color.Yellow
self.InfoLabel.Text = "Task #2 Active"
elif self.TaskState == 'Succeded':
self.TaskPanel2.BackColor = Color.Green
self.InfoLabel.Text = "Task #2 Succeded"
elif self.TaskState == 'Failed':
self.TaskPanel2.BackColor = Color.Red
self.InfoLabel.Text = "Task #2 Failed"
else:
self.TaskPanel2.BackColor = SystemColors.ControlDark
self.RedCircleLocationX = 190
self.RedCircleLocationY = 65
self.PictureBox.Invalidate()
else:
self.TaskPanel2.BackColor = SystemColors.ControlDark
if self.TaskNumber == 3:
if self.TaskState == 'Active':
self.TaskPanel3.BackColor = Color.Yellow
self.InfoLabel.Text = "Task #3 Active"
elif self.TaskState == 'Succeded':
self.TaskPanel3.BackColor = Color.Green
self.InfoLabel.Text = "Task #3 Succeded"
elif self.TaskState == 'Failed':
self.TaskPanel3.BackColor = Color.Red
self.InfoLabel.Text = "Task #3 Failed"
else:
self.TaskPanel3.BackColor = SystemColors.ControlDark
self.RedCircleLocationX = 180
self.RedCircleLocationY = 140
self.PictureBox.Invalidate()
else:
self.TaskPanel3.BackColor = SystemColors.ControlDark
def TimerTickHandler(self, sender, args):
# Handles timer tick
#print self
self.UpdateState()
def ResizeHandler(self, sender, args):
# Handles the Resize event
self.UpdateLayout()
return StatusPanel(hwnd)
|