A Product Selection Page is created - the page is connected to an
ExternalData tool in the toolbox
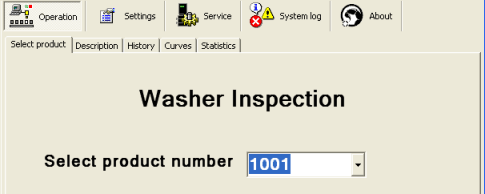
This is the GUI created by the following script example
# Central Start
# Python start script goes here
print 'Central Start'
selectProductOverlay = CreateSelectProductOverlay(GetCustomPage('Select product').handle,'ProductType')
# Central Stop
# Python stop script goes here
try:
selectProductOverlay.Dispose()
except:
pass
#
CreateSelectProductOverlay
def CreateSelectProductOverlay(hwnd,nameOfExternalData):
import CLR
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, Pen, Color, SystemColors
from CLR.System.Drawing import ContentAlignment, Font, FontStyle, Image
from CLR.System import Int32, Decimal
# Load custom assemblies
from CLR.System.Reflection import Assembly
Assembly.LoadFrom('OverlayPanel.dll')
from CLR.Tordivel import OverlayPanel
# WinForms.ComboBox connected to an ExternalData tool
class SelectProductCombo(WinForms.ComboBox):
def __init__(self):
pass
def Init(self,nameOfExternalDataTool):
self.ExternalData = nameOfExternalDataTool
self.SelectedValueChanged += self.SelectedValueChangedHandler
self.SetProductComboBox()
def SetProductComboBox(self):
plist = GetResultValue(self.ExternalData+'.Dictionary')
list = []
for element in plist:
list.append(element)
print list
list.sort()
for product in list:
self.Items.Add(str(product))
self.Text = GetValue(self.ExternalData+'.Value')
# eventhandler
def SelectedValueChangedHandler(self, sender, args):
SetValue(self.ExternalData+'.Value',self.Text)
class SelectProductOverlay(OverlayPanel):
def __init__(self,nativeParentHandleAsInt32):
# This enables the panel to follow resizing of the parent
self.Dock = WinForms.DockStyle.Fill
self.Title = "Washer Inspection"
self.LabelText = "Select product number"
self.FontName = "Microsoft Sans Serif"
# Create the profile label
self.InspectionName = WinForms.Label()
self.InspectionName.Parent = self
self.InspectionName.BorderStyle = WinForms.BorderStyle.None
self.InspectionName.Font = Font(self.FontName, 22, FontStyle.Bold)
self.InspectionName.Size = Size(300, 50)
self.InspectionName.Location= Point ((self.Width/2-self.InspectionName.Width/2),30)
self.InspectionName.Text = self.Title
self.InspectionName.TextAlign = ContentAlignment.MiddleCenter
# Create the inputLabel (after the textBox to avoid hiding)
self.SelectProductLabel = WinForms.Label()
self.SelectProductLabel.Parent = self
self.SelectProductLabel.Font = Font(self.FontName, 16, FontStyle.Bold)
self.SelectProductLabel.Size = Size(250,25)
self.SelectProductLabel.Location = Point((self.Width/2-self.SelectProductLabel.Width)/2,120)
self.SelectProductLabel.Text = self.LabelText
self.SelectProductLabel.TextAlign = ContentAlignment.MiddleCenter
# Create the select product combobox
self.SelectProduct = SelectProductCombo()
self.SelectProduct.Parent = self
self.SelectProduct.Size = Size(150,30)
self.SelectProduct.Location = Point((3*self.Width/2-self.SelectProduct.Width)/2,120)
self.SelectProduct.Font= Font(self.FontName, 16, FontStyle.Bold)
self.SelectProduct.Init(nameOfExternalData)
# Register event handler for Resize
self.Resize += self.ResizeHandler
def ResizeHandler(self, sender, args):
# Handles the Resize event
self.InspectionName.Location = Point((self.Width/2-self.InspectionName.Width/2),30)
self.SelectProduct.Location = Point(self.Width/2+5,120)
self.SelectProductLabel.Location = Point(self.SelectProduct.Location.X-(self.SelectProductLabel.Width+10),120)
return SelectProductOverlay(hwnd)
|