The SimpleTextPrint component created by Tordivel
makes it easy to print simple text documents of multiple pages in a
non-blocking manner. The component is used in a very simple
manner by using the default setting, but is also quite complete
regarding print preview, progress, font and tab size.
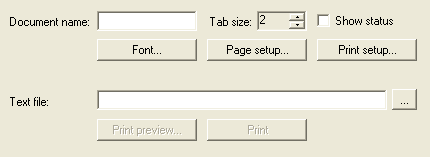
This is the GUI created by the following script
example.
A corresponding profile Dotnet - SimpleTextPrint is available on
Scorpion Support Web.
# Python start script
goes here
print 'Central Start'
import CLR
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, ContentAlignment, Font
from CLR.System import Decimal
from CLR.System.IO import StreamReader, File
# Load custom assemblies
from CLR.System.Reflection import Assembly
scorpionDir = GetValue('System.ScorpionDir')
Assembly.LoadFrom(scorpionDir + r'\OverlayPanel.dll')
from CLR.Tordivel import OverlayPanel
# Custom .NET components should be placed in DotNet folder in profile
path
profile = GetValue('System.Profile')
Assembly.LoadFrom(profile + r'\DotNet\SimpleTextPrint.dll')
from CLR.Tordivel import SimpleTextPrint
class ResultPanelOverlay(OverlayPanel):
def __init__(self,nativeParentHandleAsInt32):
self.Dock = WinForms.DockStyle.Fill
# Create the SimpleTextPrint component
self.simpleTextPrint = SimpleTextPrint()
# Create the documentNameTextBox
self.documentNameTextBox = WinForms.TextBox()
self.documentNameTextBox.Parent = self
self.documentNameTextBox.Size = Size(100,25)
self.documentNameTextBox.Location = Point(100,12)
# Register event handler for documentNameTextBox.TextChanged
self.documentNameTextBox.TextChanged +=
self.documentNameTextBox_TextChanged_Handler
# Create the documentNameLabel (after the documentNameTextBox
to avoid hiding)
self.documentNameLabel = WinForms.Label()
self.documentNameLabel.Parent = self
self.documentNameLabel.Size = Size(120,25)
self.documentNameLabel.Location = Point(10,10)
self.documentNameLabel.Text = "Document name:"
self.documentNameLabel.TextAlign =
ContentAlignment.MiddleLeft
# Create the tabSizeUpDown
self.tabSizeUpDown = WinForms.NumericUpDown()
self.tabSizeUpDown.Parent = self
self.tabSizeUpDown.Size = Size(50,25)
self.tabSizeUpDown.Location = Point(260,12)
self.tabSizeUpDown.Minimum = Decimal(0)
self.tabSizeUpDown.Maximum = Decimal(100)
self.tabSizeUpDown.Value =
Decimal(self.simpleTextPrint.TabSize)
self.tabSizeUpDown.ReadOnly = 1
# Register event handler for tabSizeUpDown.ValueChanged
self.tabSizeUpDown.ValueChanged +=
self.tabSizeUpDown_ValueChangedHandler
# Create the tabSizeLabel (after the tabSizeUpDown to avoid
hiding)
self.tabSizeLabel = WinForms.Label()
self.tabSizeLabel.Parent = self
self.tabSizeLabel.Size = Size(100,25)
self.tabSizeLabel.Location = Point(210,10)
self.tabSizeLabel.Text = "Tab size:"
self.tabSizeLabel.TextAlign = ContentAlignment.MiddleLeft
# Create the showStatusCheckBox
self.showStatusCheckBox = WinForms.CheckBox()
self.showStatusCheckBox.Parent = self
self.showStatusCheckBox.Size = Size(100,25)
self.showStatusCheckBox.Location = Point(320,10)
self.showStatusCheckBox.Text = "Show status"
self.showStatusCheckBox.Checked =
self.simpleTextPrint.ShowStatus
# Register event handler for
showStatusCheckBox.CheckedChanged
self.showStatusCheckBox.CheckedChanged +=
self.showStatusCheckBox_CheckedChanged_Handler
# Create the fontButton
self.fontButton = WinForms.Button()
self.fontButton.Parent = self
self.fontButton.Size = Size(100,22)
self.fontButton.Location = Point(100,40)
self.fontButton.Text = "Font..."
# Register event handler for fontButton.Click
self.fontButton.Click += self.fontButton_Click_Handler
# Create the pageSetupButton
self.pageSetupButton = WinForms.Button()
self.pageSetupButton.Parent = self
self.pageSetupButton.Size = Size(100,22)
self.pageSetupButton.Location = Point(210,40)
self.pageSetupButton.Text = "Page setup..."
# Register event handler for pageSetupButton.Click
self.pageSetupButton.Click +=
self.pageSetupButton_Click_Handler
# Create the printSetupButton
self.printSetupButton = WinForms.Button()
self.printSetupButton.Parent = self
self.printSetupButton.Size = Size(100,22)
self.printSetupButton.Location = Point(320,40)
self.printSetupButton.Text = "Print setup..."
# Register event handler for printSetupButton.Click
self.printSetupButton.Click +=
self.printSetupButton_Click_Handler
# Create the textFilePathNameTextBox
self.textFilePathNameTextBox = WinForms.TextBox()
self.textFilePathNameTextBox.Parent = self
self.textFilePathNameTextBox.Size = Size(290,25)
self.textFilePathNameTextBox.Location = Point(100,91)
# Register event handler for
textFilePathNameTextBox.TextChanged
self.textFilePathNameTextBox.TextChanged +=
self.textFilePathNameTextBox_TextChanged_Handler
# Create the textFilePathNameLabel (after the
textFilePathNameTextBox to avoid hiding)
self.textFilePathNameLabel = WinForms.Label()
self.textFilePathNameLabel.Parent = self
self.textFilePathNameLabel.Size = Size(120,25)
self.textFilePathNameLabel.Location = Point(10,90)
self.textFilePathNameLabel.Text = "Text file:"
self.textFilePathNameLabel.TextAlign =
ContentAlignment.MiddleLeft
# Create the browseButton
self.browseButton = WinForms.Button()
self.browseButton.Parent = self
self.browseButton.Size = Size(25,21)
self.browseButton.Location = Point(395,90)
self.browseButton.Text = "..."
# Register event handler for browseButton.Click
self.browseButton.Click += self.browseButton_Click_Handler
# Create the printPreviewButton
self.printPreviewButton = WinForms.Button()
self.printPreviewButton.Parent = self
self.printPreviewButton.Size = Size(100,22)
self.printPreviewButton.Location = Point(100,120)
self.printPreviewButton.Text = "Print preview..."
self.printPreviewButton.Enabled = 0
# Register event handler for printPreviewButton.Click
self.printPreviewButton.Click +=
self.printPreviewButton_Click_Handler
# Create the printButton
self.printButton = WinForms.Button()
self.printButton.Parent = self
self.printButton.Size = Size(100,22)
self.printButton.Location = Point(210,120)
self.printButton.Text = "Print"
self.printButton.Enabled = 0
# Register event handler for printButton.Click
self.printButton.Click += self.printButton_Click_Handler
def documentNameTextBox_TextChanged_Handler(self, sender, args):
# Handles the documentNameTextBox TextChanged event
self.simpleTextPrint.PrintDocument.DocumentName =
self.documentNameTextBox.Text
def showStatusCheckBox_CheckedChanged_Handler(self, sender, args):
# Handles the showStatusCheckBox CheckedChanged event
self.simpleTextPrint.ShowStatus =
self.showStatusCheckBox.Checked
def tabSizeUpDown_ValueChangedHandler(self, sender, args):
# Handles the tabSizeUpDown ValueChanged event
self.simpleTextPrint.TabSize =
Decimal.ToInt32(self.tabSizeUpDown.Value)
def fontButton_Click_Handler(self, sender, args):
# Handles the fontButton Click event
fontDialog = WinForms.FontDialog()
fontDialog.Font = self.simpleTextPrint.PrintFont
if (fontDialog.ShowDialog(self) == WinForms.DialogResult.OK):
self.simpleTextPrint.PrintFont = fontDialog.Font
def pageSetupButton_Click_Handler(self, sender, args):
# Handles the pageSetupButton Click event
self.simpleTextPrint.ShowPageSetupDialog(self)
def printSetupButton_Click_Handler(self, sender, args):
# Handles the printSetupButton Click event
self.simpleTextPrint.ShowPrintDialog(self)
def textFilePathNameTextBox_TextChanged_Handler(self, sender, args):
# Handles the textFilePathNameTextBox TextChanged event
if File.Exists(self.textFilePathNameTextBox.Text):
self.printButton.Enabled = 1
self.printPreviewButton.Enabled = 1
else:
self.printButton.Enabled = 0
self.printPreviewButton.Enabled = 0
def browseButton_Click_Handler(self, sender, args):
# Handles the browseButton Click event
openFileDialog = WinForms.OpenFileDialog()
openFileDialog.Filter = "Text Files (*.txt)|*.txt|All Files
(*.*)|*.*||"
if (openFileDialog.ShowDialog() == WinForms.DialogResult.OK):
self.textFilePathNameTextBox.Text =
openFileDialog.FileName
def printPreviewButton_Click_Handler(self, sender, args):
# Handles the printPreviewButton Click event
self.simpleTextPrint.PrintPreview(self,StreamReader(self.textFilePathNameTextBox.Text))
def printButton_Click_Handler(self, sender, args):
# Handles the printButton Click event
self.simpleTextPrint.Print(StreamReader(self.textFilePathNameTextBox.Text))
# try to dispose existing global resultPanelOverlay instance
try:
resultPanelOverlay.Dispose()
except:
print 'Dispose Failed'
# Create global instance of resultPanelOverlay
resultPanelOverlay = ResultPanelOverlay(GetResultPanel().handle)
|