Example 1: Add/replace a template by marking an area in main picture
tool = GetTool('TF3')
tool.executeCmd('Set','Object=Template;Name=A;source=clipboard') #from clipboard
tool.executeCmd('Set','Object=Template;Name=A;value={(190;360)(190;420)(230;420)(230;360)}') #absolute values
This will extract an area (rectangle) surrounding the marked points in
the main image and use the subimage within this area as a new template. If
the named template already exists it is replaced, keeping current settings
as far as possible; otherwise a new template is added with default settings.
The selected area is highlighted in the main image.
The marked area must be within the tool's ROI.
Example 2: Activate/deactivate templates
tool = GetTool('TF3')
tool.executeCmd('Set','Object=Template;Name=A;Active=0')
This deactivates any templates named A.
Example 3: Activate unique templates
tool = GetTool('TF3')
tool.executeCmd('Set','Object=Template;Name=A;Active=1;Unique=1')
This activates any templates named A, and deactivates all others.
Example 4: Setting PolygonMatch configuration parameters from Script
import SPB
pm=GetTool('PM')
spb=SPB.CreateSpb(t.config)
#Get parameter values from
rcount=GetIntValue('Tf3Setup.PolygonRepeatCount')
kperc=GetFloatValue('Tf3Setup.PolygonKeepPercent')
fd=GetFloatValue('Tf3Setup.PolygonMaxFitDistance')
mp=GetFloatValue('Tf3Setup.PolygonMinMatchPercent')
val=GetBoolValue('Tf3Setup.MatchValidation')
#Set parameter values
spb.setInt('EdgeMatchCount',rcount)
spb.setFloat('KeepPercent',kperc)
spb.setFloat('FinalFitDist',fd)
spb.setFloat('MinPercent',mp)
spb.setBool('MustMatch',val)
#Update the spb
pm.config=spb.xml
Example 5: Creating a point list from tf3 match centers
def Tf3Points(name):
points = []
tf3 = GetTool(name)
count = tf3.getIntValue('Count')
for i in range(count):
x = tf3.getValue('MatchCenter['+str(int(i+1))+']_x')
y = tf3.getValue('MatchCenter['+str(int(i+1))+']_y')
points.append((x,y))
return count,points
Example 6: Calculating the distance between two points in match
center list
import math
def distance(points,index) :
x0 = points[index][0]
x1 = points[index+1][0]
y0 = points[index][1]
y1 = points[index+1][1]
return math.sqrt( (x1-x0)*(x1-x0) + (y1-y0)*(y1-y0))
Example 7: Draw distances as an image overlay
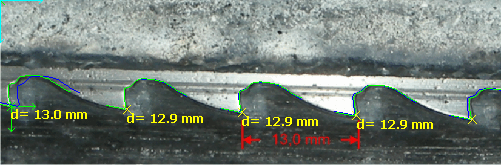
count,points = Tf3Points('tf3')
if count > 1:
dist=[]
for i in range(count-1):
d = distance(points,i)
distStr = 'd= %(d).1f mm' %vars()
DrawText('scale',points[i][0],points[int(i)][1],distStr,'yellow',10,'','',1)
dist.append(d)
Example 8: Basic Python Wrapper Class for TemplateFinder3
def CreateTf3(name):
class Tf3:
def __init__(self,name):
self.name = name
def X(self,number):
return GetFloatValue(self.name+'.MatchCenter[%d]_x' % number)
def Y(self,number):
return GetFloatValue(self.name+'.MatchCenter[%d]_y' % number)
def Angle(self,number):
return GetFloatValue(self.name+'.Angle[%d]' % number)
def SizeX(self,number):
return GetFloatValue(self.name+'.Size[%d]_x' % number)
def SizeY(self,number):
return GetFloatValue(self.name+'.Size[%d]_y' % number)
def Size(self,number):
return self.SizeX(number),self.SizeY(number)
def GetCenter(self,number):
center = GetResultValue(self.name+'.AllCenters')
return center[number-1][0],center[number-1][1]
def GetPosition(self,number):
score = self.GetScore(number)
status = self.GetStatus()
return self.X(number),self.Y(number),self.Angle(number),score,status
def SetPosition(self,number, x,y,angle):
SetFloatValue(self.name+'.MatchCenter[%d]_x' % number,x)
SetFloatValue(self.name+'.MatchCenter[%d]_y' % number,y)
SetFloatValue(self.name+'.Angle[%d]' % number,angle)
def GetScore(self,number):
return GetFloatValue(self.name+'.Score[%d]' % number)
def GetCount(self):
return GetFloatValue(self.name+'.Count')
def SetStatus(self,status):
SetIntValue(self.name+'.Status',status)
def GetStatus(self):
return GetIntValue(self.name+'.Status')
return Tf3(name)